Game Rotation
The red square can rotate:
Rotating Components
Earlier in this tutorial, the red square was able to move around on the gamearea, but it could not turn or rotate.
To rotate components, we have to change the way we draw components.
The only rotation method available for the canvas element will rotate the entire canvas:
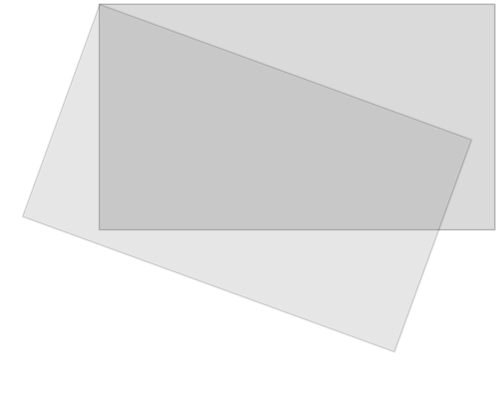
Everything else you draw on the canvas will also be rotated, not only the specific component.
That is why we have to make some changes in the update()
method:
First, we save the current canvas context object:
ctx.save();
Then we move the entire canvas to the center of the specific component, using the translate method:
ctx.translate(x, y);
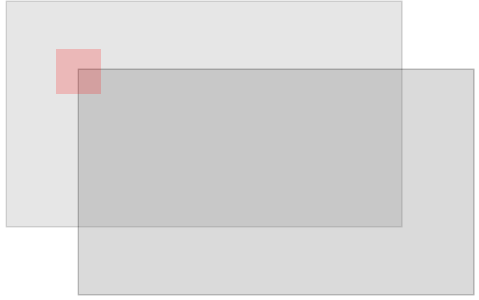
Then we perform the wanted rotation using the rotate() method:
ctx.rotate(angle);
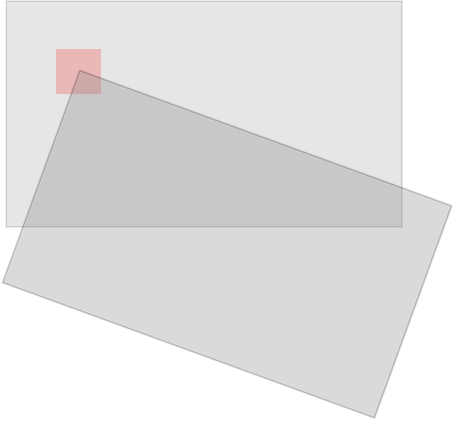
Now we are ready to draw the component onto the canvas, but now we will draw it with its center position at position 0,0 on the translated (and rotated) canvas:
ctx.fillRect(width / -2, height / -2, width, height);
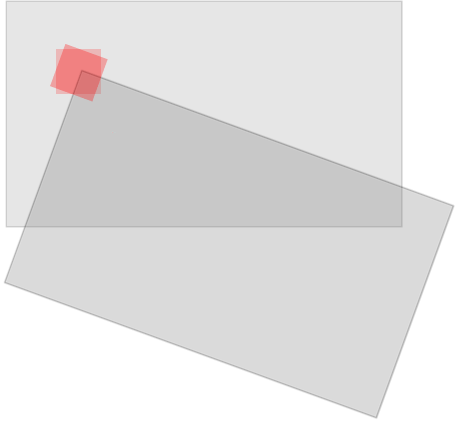
When we are finished, we must restore the context object back to its saved position, using the restore method:
ctx.restore();
The component is the only thing that is rotated:
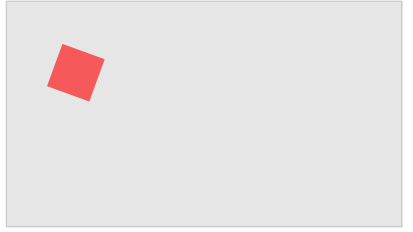
The Component Constructor
The component
constructor has a new property called angle
,
which is radian number that represents the angle of the component.
The update
method of the component
constructor is
were we draw the component, and here you can see the changes that will allow the
component to rotate:
Example
function component(width, height, color, x, y) {
this.width = width;
this.height = height;
this.angle = 0;
this.x = x;
this.y = y;
this.update = function() {
ctx = myGameArea.context;
ctx.save();
ctx.translate(this.x, this.y);
ctx.rotate(this.angle);
ctx.fillStyle = color;
ctx.fillRect(this.width / -2, this.height / -2, this.width, this.height);
ctx.restore();
}
}
function updateGameArea() {
myGameArea.clear();
myGamePiece.angle += 1 * Math.PI / 180;
myGamePiece.update();
}
Try it Yourself »