XML DOM Tutorial
XML DOM
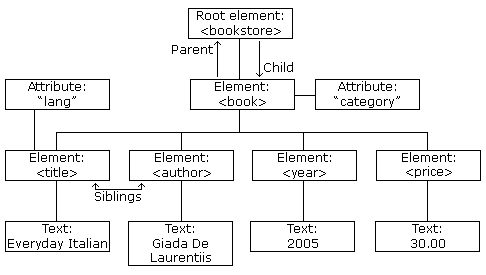
What is the DOM?
The DOM defines a standard for accessing and manipulating documents:
The HTML DOM defines a standard way for accessing and manipulating HTML documents. It presents an HTML document as a tree-structure.
The XML DOM defines a standard way for accessing and manipulating XML documents. It presents an XML document as a tree-structure.
Understanding the DOM is a must for anyone working with HTML or XML.
The HTML DOM
All HTML elements can be accessed through the HTML DOM.
This example changes the value of an HTML element with id="demo":
Example
<h1 id="demo">This is a Heading</h1>
<script>
document.getElementById("demo").innerHTML = "Hello World!";
</script>
Try it Yourself »
This example changes the value of the first <h1> element in an HTML document:
Example
<h1>This is a Heading</h1>
<h1>This is a Heading</h1>
<script>
document.getElementsByTagName("h1")[0].innerHTML = "Hello World!";
</script>
Try it Yourself »
Note: Even if the HTML document contains only ONE <h1> element you still have to specify the array index [0], because the getElementsByTagName() method always returns an array.
You can learn a lot more about the HTML DOM in our JavaScript tutorial.
The XML DOM
All XML elements can be accessed through the XML DOM.
The XML DOM is:
- A standard object model for XML
- A standard programming interface for XML
- Platform- and language-independent
- A W3C standard
In other words: The XML DOM is a standard for how to get, change, add, or delete XML elements.
Get the Value of an XML Element
This code retrieves the text value of the first <title> element in an XML document:
Example
txt = xmlDoc.getElementsByTagName("title")[0].childNodes[0].nodeValue;
Loading an XML File
The XML file used in the examples below is books.xml.
This example reads "books.xml" into xmlDoc and retrieves the text value of the first <title> element in books.xml:
Example
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
myFunction(this);
}
};
xhttp.open("GET", "books.xml", true);
xhttp.send();
function myFunction(xml) {
var xmlDoc = xml.responseXML;
document.getElementById("demo").innerHTML =
xmlDoc.getElementsByTagName("title")[0].childNodes[0].nodeValue;
}
</script>
</body>
</html>
Try it Yourself »
Example Explained
- xmlDoc - the XML DOM object created by the parser.
- getElementsByTagName("title")[0] - get the first <title> element
- childNodes[0] - the first child of the <title> element (the text node)
- nodeValue - the value of the node (the text itself)
Loading an XML String
This example loads a text string into an XML DOM object, and extracts the info from it with JavaScript:
Example
<html>
<body>
<p id="demo"></p>
<script>
var text, parser,
xmlDoc;
text = "<bookstore><book>" +
"<title>Everyday
Italian</title>" +
"<author>Giada De Laurentiis</author>" +
"<year>2005</year>" +
"</book></bookstore>";
parser = new DOMParser();
xmlDoc = parser.parseFromString(text,"text/xml");
document.getElementById("demo").innerHTML =
xmlDoc.getElementsByTagName("title")[0].childNodes[0].nodeValue;
</script>
</body>
</html>
Try it Yourself »
Programming Interface
The DOM models XML as a set of node objects. The nodes can be accessed with JavaScript or other programming languages. In this tutorial we use JavaScript.
The programming interface to the DOM is defined by a set standard properties and methods.
Properties are often referred to as something that is (i.e. nodename is "book").
Methods are often referred to as something that is done (i.e. delete "book").
XML DOM Properties
These are some typical DOM properties:
- x.nodeName - the name of x
- x.nodeValue - the value of x
- x.parentNode - the parent node of x
- x.childNodes - the child nodes of x
- x.attributes - the attributes nodes of x
Note: In the list above, x is a node object.
XML DOM Methods
- x.getElementsByTagName(name) - get all elements with a specified tag name
- x.appendChild(node) - insert a child node to x
- x.removeChild(node) - remove a child node from x
Note: In the list above, x is a node object.